1. What is a Breakpoint? Why is it Useful?
A breakpoint is a marker set in your code to pause execution at a specific point. It allows you to inspect the state of variables, control flow, and data, which is especially useful for debugging issues in your JavaScript code.
Why is it Useful?
-
Helps pinpoint issues: By pausing execution at key points, breakpoints help identify where things go wrong in the flow of your program.
-
Inspect variables: You can inspect variables and the call stack at a specific point, which helps track how data changes during execution.
-
Step through code: You can step through your code line-by-line, helping you understand how different parts of your code interact and execute.
2. When Are Breakpoints Useful?
Breakpoints are especially useful when you:
-
Encounter hard-to-find bugs: Breakpoints allow you to pause and inspect the program at critical points, making it easier to identify hidden issues.
-
Want to understand the flow of your code: If you're unsure how your code is executing or interacting with different parts, breakpoints help clarify that by letting you step through the code.
-
Need to check variable states: If a function is producing unexpected results, you can use breakpoints to inspect the state of the variables at any given point.
🎥 Video: Breakpoints and logpoints by Chrome for Developers
3. How to Open the Sources Tab in DevTools and Set a Breakpoint
Opening the Sources Tab
- Open Chrome Developer Tools by pressing
Ctrl + Shift + I
(Windows/Linux) or Cmd + Option + I
(Mac).
- Go to the Sources tab where you'll find the files loaded for your web page, including JavaScript files.
Setting a Breakpoint
- In the Sources tab, find and open the JavaScript file where you want to set a breakpoint.
- Scroll to the line of code where you want to pause execution.
- Click on the line number to set a breakpoint. A blue marker will appear indicating that the breakpoint is set.
- Once the breakpoint is set, you can refresh the page or trigger the action that runs the code, and the debugger will pause execution at the breakpoint.
🎥 Tutorial: Walk through this Debugging JavaScript with Breakpoints Tutorial for a step-by-step guide.

4. How to Use the Debugger in VSCode
Using the Debugger in VSCode
- Open your project in VSCode.
- Set up a launch configuration for your app. Go to the Run menu and select Add Configuration. Choose a configuration that matches your environment (for example, Node.js for backend or Chrome for front-end).
- To start debugging, go to the Run and Debug pane and click Start Debugging or press
F5
. This will launch the debugging session.
- Set breakpoints directly in your code by clicking next to the line numbers in VSCode.
- The debugger will pause at breakpoints, allowing you to inspect variables, step through your code, and more. You can use the Debug Console to interact with your code while debugging.
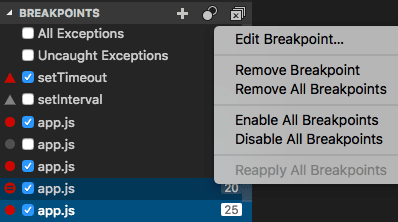
5. Types of Breakpoints & When to Use Them
Line Breakpoint – Pausing Execution at a Specific Line
A line breakpoint pauses execution at a specific line of code. This is the most basic type of breakpoint and is useful when you want to inspect what happens at a particular point.
-
Use Case: You suspect that a specific line in a function is causing an issue or you want to see how variables are updated at that point.
Conditional Breakpoint – Pauses Execution If a Condition Is Met
A conditional breakpoint pauses the code only when a certain condition is met (e.g., when a variable reaches a specific value). This is useful if the issue occurs intermittently or only under certain conditions.
-
Use Case: You only want to pause the code when a variable reaches a specific value or when a particular condition is true.
How to Set: Right-click on a line number where you set the breakpoint, and select Add Conditional Breakpoint. Enter a condition like x === 10
to pause execution when x
equals 10.

DOM Breakpoint – Pauses When an Element Changes in the DOM
A DOM breakpoint pauses execution when a specific element in the DOM changes. This is useful for debugging issues related to the dynamic changes in the DOM (e.g., element removal or attribute changes).
-
Use Case: You want to inspect the code when an element is added, removed, or modified in the DOM.
How to Set: In the Elements tab of DevTools, right-click on the element you want to track, and choose Break on → Subtree modifications, Attribute modifications, or Node removal.

Fetch Breakpoint – Pauses When an API Request is Made
A fetch breakpoint pauses execution when an API request (like a fetch
or XHR
request) is made. This helps debug issues related to network requests or API calls.
-
Use Case: You need to inspect when an API request is triggered and view the request/response details.
How to Set: Go to the Sources tab → XHR/fetch Breakpoints panel → Add a new breakpoint by specifying the URL or endpoint of the API request.

Event Listener Breakpoint – Pauses When an Event Occurs
An event listener breakpoint pauses execution when a specified event occurs, such as click
, keydown
, or submit
.
-
Use Case: You want to inspect the state of your application when a user interacts with it (e.g., clicking a button).
How to Set: In the Sources tab, go to the Event Listener Breakpoints panel. Expand the events category you are interested in (like Mouse, Keyboard, Form, etc.), and check the event type you want to track.

By mastering breakpoints, you'll have a powerful tool at your disposal for understanding and fixing issues in your JavaScript code. Whether you're tracking down a bug, optimizing performance, or just learning how your code works, breakpoints provide an invaluable way to step through execution and inspect your code in action.